Illustrating the Blockchain via SteemJs – Blocks are Chain
- Time:2020-09-09 13:08:38
- Class:Weblog
- Read:28
Blockchain is a fancy word, and not many people understand it. If you want to explain this to your GF/partner, what would be the most simple way?
Right, blocks are chained in one way, as time goes on, in particular for STEEM blockchain, every 3 seconds, we append a new block to the end of the current chain.
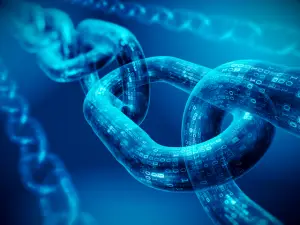
blockchain
We need a way to tell if the current block can be attached (we can’t just produce a random block and force appending it). For each block, we will have a unique block ID, and we will have a previous field that match the previous Block ID. The first block on steem blockchain has previous set to 0000000000000000000000000000000000000000. We might also notice that there are fewer transactions/data stored in the first few blocks.
Run this in SteemJS
1 2 3 4 | const blockNum = 1; steem.api.getBlock(blockNum, function(err, result) { log(result); }); |
const blockNum = 1; steem.api.getBlock(blockNum, function(err, result) { log(result); });
You will see the block_id and previous value of the first block.
1 | {"previous":"0000000000000000000000000000000000000000","timestamp":"2016-03-24T16:05:00","witness":"initminer","transaction_merkle_root":"0000000000000000000000000000000000000000","extensions":[],"witness_signature":"204f8ad56a8f5cf722a02b035a61b500aa59b9519b2c33c77a80c0a714680a5a5a7a340d909d19996613c5e4ae92146b9add8a7a663eef37d837ef881477313043","transactions":[],"block_id":"0000000109833ce528d5bbfb3f6225b39ee10086","signing_key":"STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX","transaction_ids":[]} |
{"previous":"0000000000000000000000000000000000000000","timestamp":"2016-03-24T16:05:00","witness":"initminer","transaction_merkle_root":"0000000000000000000000000000000000000000","extensions":[],"witness_signature":"204f8ad56a8f5cf722a02b035a61b500aa59b9519b2c33c77a80c0a714680a5a5a7a340d909d19996613c5e4ae92146b9add8a7a663eef37d837ef881477313043","transactions":[],"block_id":"0000000109833ce528d5bbfb3f6225b39ee10086","signing_key":"STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX","transaction_ids":[]}
And the second block gives:
1 | {"previous":"0000000109833ce528d5bbfb3f6225b39ee10086","timestamp":"2016-03-24T16:05:36","witness":"initminer","transaction_merkle_root":"0000000000000000000000000000000000000000","extensions":[],"witness_signature":"1f3e85ab301a600f391f11e859240f090a9404f8ebf0bf98df58eb17f455156e2d16e1dcfc621acb3a7acbedc86b6d2560fdd87ce5709e80fa333a2bbb92966df3","transactions":[],"block_id":"00000002ed04e3c3def0238f693931ee7eebbdf1","signing_key":"STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX","transaction_ids":[]} |
{"previous":"0000000109833ce528d5bbfb3f6225b39ee10086","timestamp":"2016-03-24T16:05:36","witness":"initminer","transaction_merkle_root":"0000000000000000000000000000000000000000","extensions":[],"witness_signature":"1f3e85ab301a600f391f11e859240f090a9404f8ebf0bf98df58eb17f455156e2d16e1dcfc621acb3a7acbedc86b6d2560fdd87ce5709e80fa333a2bbb92966df3","transactions":[],"block_id":"00000002ed04e3c3def0238f693931ee7eebbdf1","signing_key":"STM8GC13uCZbP44HzMLV6zPZGwVQ8Nt4Kji8PapsPiNq1BK153XTX","transaction_ids":[]}
As you can see, the previous value is the same as the block_id for the Block 1. This goes on and on. Let’s print the first 10 blocks’ previous and block_id:
Run the Code in SteemJS
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | function getBlock(blockNum) { return new Promise((resolve, reject) => { steem.api.getBlock(blockNum, function(err, result) { if (err) reject(err); resolve({ block: blockNum, previous: result.previous, block_id: result.block_id, }); }); }); } (async function() { for (let i = 1; i <= 10; ++ i) { log(await getBlock(i)); } })(); |
function getBlock(blockNum) { return new Promise((resolve, reject) => { steem.api.getBlock(blockNum, function(err, result) { if (err) reject(err); resolve({ block: blockNum, previous: result.previous, block_id: result.block_id, }); }); }); } (async function() { for (let i = 1; i <= 10; ++ i) { log(await getBlock(i)); } })();
And you will see the rule applies. In fact, this is the fundamental rule of the blockchain – the rule to chain the blocks.
1 2 3 4 5 6 7 8 9 10 | {"block":1,"previous":"0000000000000000000000000000000000000000","block_id":"0000000109833ce528d5bbfb3f6225b39ee10086"} {"block":2,"previous":"0000000109833ce528d5bbfb3f6225b39ee10086","block_id":"00000002ed04e3c3def0238f693931ee7eebbdf1"} {"block":3,"previous":"00000002ed04e3c3def0238f693931ee7eebbdf1","block_id":"000000035b094a812646289c622dba0ba67d1ffe"} {"block":4,"previous":"000000035b094a812646289c622dba0ba67d1ffe","block_id":"00000004f9de0cfeb08c9d7d9d1fe536d902dc4a"} {"block":5,"previous":"00000004f9de0cfeb08c9d7d9d1fe536d902dc4a","block_id":"00000005014b5562a1133070d8bee536de615329"} {"block":6,"previous":"00000005014b5562a1133070d8bee536de615329","block_id":"00000006e323e35687e160b8aec86f1e56d4c902"} {"block":7,"previous":"00000006e323e35687e160b8aec86f1e56d4c902","block_id":"000000079ff02a2dea6c4d9a27f752233d4a66b4"} {"block":8,"previous":"000000079ff02a2dea6c4d9a27f752233d4a66b4","block_id":"000000084f957cc170a27c8330293a3343f82c23"} {"block":9,"previous":"000000084f957cc170a27c8330293a3343f82c23","block_id":"00000009f35198cfd8a866868538bed3482d61a4"} {"block":10,"previous":"00000009f35198cfd8a866868538bed3482d61a4","block_id":"0000000aae44a2f4d57170dab16fb1619f9e1d0e"} |
{"block":1,"previous":"0000000000000000000000000000000000000000","block_id":"0000000109833ce528d5bbfb3f6225b39ee10086"} {"block":2,"previous":"0000000109833ce528d5bbfb3f6225b39ee10086","block_id":"00000002ed04e3c3def0238f693931ee7eebbdf1"} {"block":3,"previous":"00000002ed04e3c3def0238f693931ee7eebbdf1","block_id":"000000035b094a812646289c622dba0ba67d1ffe"} {"block":4,"previous":"000000035b094a812646289c622dba0ba67d1ffe","block_id":"00000004f9de0cfeb08c9d7d9d1fe536d902dc4a"} {"block":5,"previous":"00000004f9de0cfeb08c9d7d9d1fe536d902dc4a","block_id":"00000005014b5562a1133070d8bee536de615329"} {"block":6,"previous":"00000005014b5562a1133070d8bee536de615329","block_id":"00000006e323e35687e160b8aec86f1e56d4c902"} {"block":7,"previous":"00000006e323e35687e160b8aec86f1e56d4c902","block_id":"000000079ff02a2dea6c4d9a27f752233d4a66b4"} {"block":8,"previous":"000000079ff02a2dea6c4d9a27f752233d4a66b4","block_id":"000000084f957cc170a27c8330293a3343f82c23"} {"block":9,"previous":"000000084f957cc170a27c8330293a3343f82c23","block_id":"00000009f35198cfd8a866868538bed3482d61a4"} {"block":10,"previous":"00000009f35198cfd8a866868538bed3482d61a4","block_id":"0000000aae44a2f4d57170dab16fb1619f9e1d0e"}
Steem Blockchain is a public database. Every 3 seconds, one witness helps to package operations (comment, vote, transfer etc) into a block, seal it with the signing key, and finally push to the chain for a small reward.
As the blockchain gets bigger and bigger, it takes enormous efforts to alter the chain, as you will need to modify every prior blocks.
–EOF (The Ultimate Computing & Technology Blog) —
Recommend:How to Blog Like Shakespeare
Depth First Search Algorithm to Delete Insufficient Nodes in Roo
C/C++ Program to Compute the Angle Between Hands of a Clock
What Should Your Anti Virus Software Have in Order to Be Effecti
The Importance of SEO and How They Improve the Number of Your Cl
Learn The Basics Of Google My Business
Iterative and Recursion Algorithms to Compute the Number of Step
How to Check if a DLL or EXE is 32-bit or 64-bit (x86 or x64) us
C++: How to Iterate the Elements over the Sets (set, unordered_s
How to Iterate over the Items in Java’s Map (HashMap, Hash
- Comment list
-
- Comment add