Subtract the Product and Sum of Digits of an Integer
- Time:2020-09-15 16:10:27
- Class:Weblog
- Read:31
Given an integer number n, return the difference between the product of its digits and the sum of its digits.
Example 1:
Input: n = 234
Output: 15
Explanation:
Product of digits = 2 * 3 * 4 = 24
Sum of digits = 2 + 3 + 4 = 9
Result = 24 – 9 = 15Example 2:
Input: n = 4421
Output: 21
Explanation:
Product of digits = 4 * 4 * 2 * 1 = 32
Sum of digits = 4 + 4 + 2 + 1 = 11
Result = 32 – 11 = 21Constraints:
1 <= n <= 10^5Hints:
How to compute all digits of the number?
Use modulus operator (%) to compute the last digit.
Generalise modulus operator idea to compute all digits.
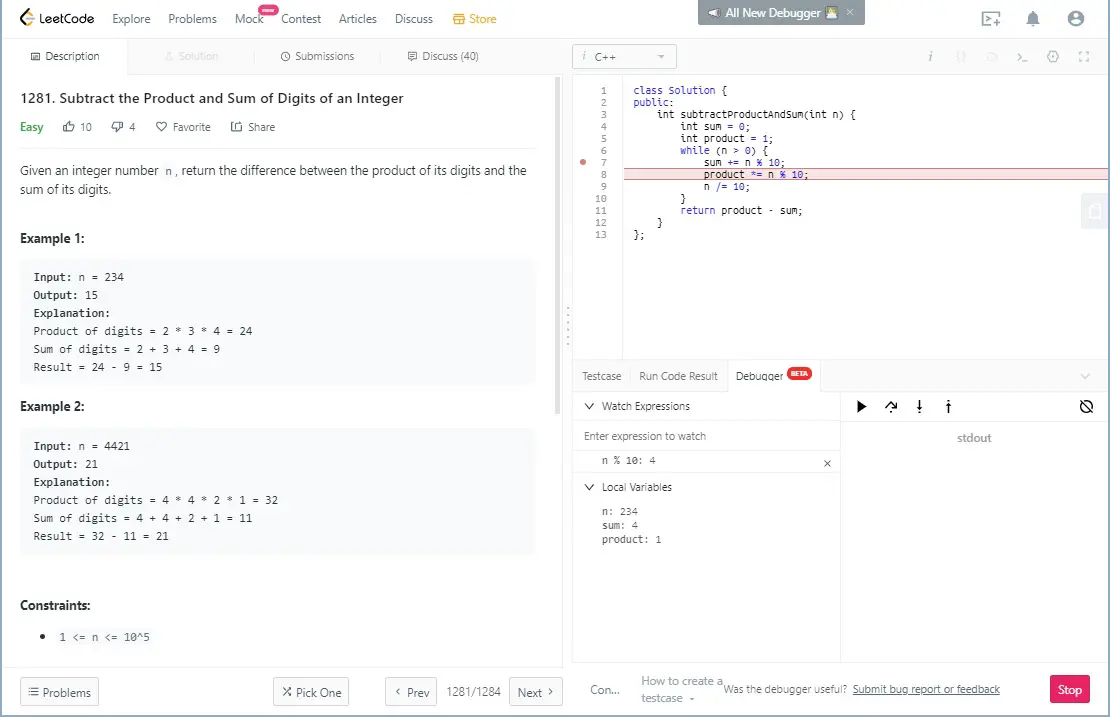
leetcode-debugger
To get the digits of a integer, we can iteratedly divide the integer by ten, and retrieve the right-most digit by using modulous operator (%). The time complexity is O(lgN).
1 2 3 4 5 6 7 8 9 10 11 12 13 | class Solution { public: int subtractProductAndSum(int n) { int sum = 0; int product = 1; while (n > 0) { sum += n % 10; product *= n % 10; n /= 10; } return product - sum; } }; |
class Solution { public: int subtractProductAndSum(int n) { int sum = 0; int product = 1; while (n > 0) { sum += n % 10; product *= n % 10; n /= 10; } return product - sum; } };
The C++ code above requires O(1) constant space. And in Python, you could convert the integer into string, join them with a space, then split them into array of digit strings, finally convert them into list of digits by using the map function.
1 2 3 4 5 6 7 | from operator import mul from functools import reduce class Solution: def subtractProductAndSum(self, n: int) -> int: temp = list(map(int, ' '.join(str(n)).split())) return reduce(mul, temp, 1) - sum(temp) |
from operator import mul from functools import reduce class Solution: def subtractProductAndSum(self, n: int) -> int: temp = list(map(int, ' '.join(str(n)).split())) return reduce(mul, temp, 1) - sum(temp)
Then, we can compute the produce by using the reduce function, with the mul operator. The sum of digits can be just obtained via the sum() function.
–EOF (The Ultimate Computing & Technology Blog) —
Recommend:DFS and BFS Algorithm to Find Numbers With Same Consecutive Diff
How to Remove Items/Entries with Specific Values from Map/HashMa
Find the Real Root of 4^x + 6^x = 9^x
Depth First Search (Backtracking) Algorithm to Solve a Sudoku Ga
Using Bitmasking Algorithm to Compute the Combinations of an Arr
Flashing the BIOS of HPZ800 Server to 3.61 Rev.A
Algorithm to Sum The Fibonacci Numbers
How to Adapt Your Blog to Increasing Zero-Click Searches
Understanding Marketing Automation & Its Perks for Your Busi
Adobe Flash Player Nears its EOL, Will this Affect your Blog?
- Comment list
-
- Comment add